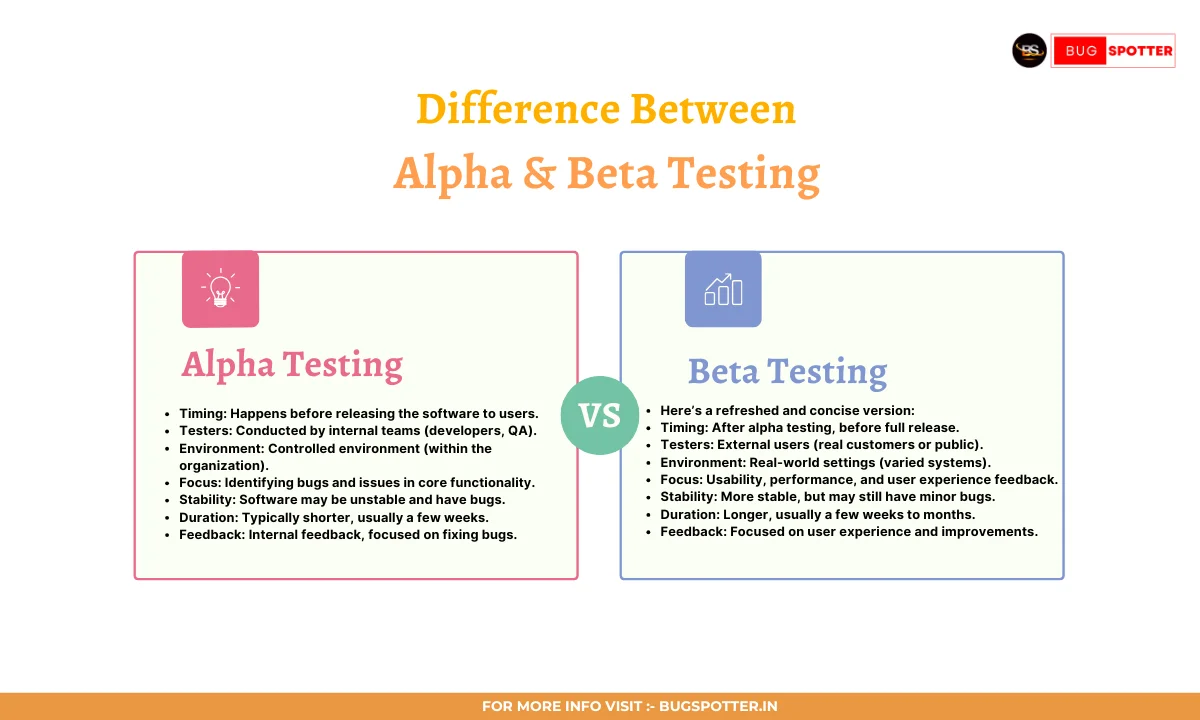
Selenium is an open-source tool for automating web browsers. It allows the automation of web applications to ensure their functionality. It supports multiple programming languages, including Java, Python, and C#.
Â
Selenium WebDriver, Selenium IDE, Selenium Grid, Selenium RC. WebDriver is used for automation, IDE is a browser-based tool for recording and playback, Grid is for parallel test execution, and RC (now deprecated) was used for remote test execution.
Â
Selenium 2.0 combines WebDriver and RC, while Selenium 3.0 drops RC in favor of WebDriver and focuses solely on browser automation. Selenium 3.0 has better support for modern browsers and is more stable.
Â
WebDriver is the main API for controlling browsers in Selenium. It interacts directly with the browser, simulating user actions such as clicking buttons, entering text, and navigating through web pages.
Â
Selenium is open-source, supports multiple browsers and programming languages, and runs on different platforms (Windows, Linux, macOS). QTP (QuickTest Professional) is a paid tool, primarily used for functional testing on Windows and only supports VBScript.
Â
Implicit Wait, Explicit Wait, Fluent Wait. Implicit Wait waits for a specified time before throwing an exception, Explicit Wait is used for waiting on specific conditions, and Fluent Wait allows custom polling intervals and exception handling.
Â
Locators are strategies used to identify web elements on a page, such as ID, Name, Class Name, XPath, CSS Selector, Tag Name, Link Text, and Partial Link Text.
Â
findElement()
and findElements()
?findElement()
returns the first matching element, throwing an exception if none are found. findElements()
returns a list of all matching elements, or an empty list if no elements are found.
Â
driver.get()
and driver.navigate().to()
?driver.get()
loads a URL and waits for the page to load completely, while driver.navigate().to()
can be used for navigation, allowing you to visit a URL without waiting for the page to fully load.
Â
XPath is a language used to navigate through elements in XML/HTML documents. In Selenium, it’s used to locate elements using their structure or attributes (e.g., //input[@id='username']
).
Â
Absolute XPath starts from the root element (/html/body/...
), while Relative XPath starts from any element and is more flexible (//div[@class='example']
).
Â
POM is a design pattern that creates a separate class for each web page. This promotes code reusability and maintainability by keeping UI operations separate from the test logic.
13. How does Selenium WebDriver interact with browsers?
WebDriver interacts with the browser by communicating with its native APIs, allowing it to perform browser actions directly, like navigating, clicking, and filling forms.
Â
14.How do you handle dynamic elements in Selenium?
Use dynamic locators (e.g., XPath with contains()
, starts-with()
) or Explicit Waits to wait for elements to appear, ensuring stability when dealing with elements that change frequently.
Â
15.What are the different ways to handle multiple windows in Selenium?
Use getWindowHandles()
to get all window handles and switchTo().window()
to switch between them. This helps in managing pop-up windows or multiple tabs.
Â
16.How can you perform mouse actions in Selenium?
Use the Actions class to simulate mouse actions like hover (moveToElement()
), right-click (contextClick()
), and drag-and-drop (dragAndDrop()
).
Â
17.What is the difference between driver.quit()
and driver.close()
?
driver.quit()
closes all browser windows and ends the WebDriver session, while driver.close()
only closes the current browser window controlled by WebDriver.
Â
18.How do you take a screenshot in Selenium?
Use the TakesScreenshot
interface with getScreenshotAs()
method to capture the screenshot and store it as a file. This is useful for debugging failed tests.
Â
19.How do you perform file upload in Selenium?
You can use sendKeys()
to simulate entering a file path in an input field of type file (e.g., <input type="file">
), which triggers a file upload dialog.
Â
20.How do you handle alerts in Selenium?
Use the Alert
interface for accepting, dismissing, retrieving text, or sending text to alerts with methods like accept()
, dismiss()
, getText()
, and sendKeys()
.
Â
21.How do you perform keyboard actions in Selenium?
Use the Actions class to simulate keyboard actions such as pressing keys (keyDown()
), releasing keys (keyUp()
), and typing text (sendKeys()
).
Â
22.What is the role of @FindBy
annotation in Page Object Model (POM)?
@FindBy
is used to locate elements in a page class. It simplifies the process of element initialization, making the code more readable and maintainable.
23.What is Selenium Grid and how does it work?
Selenium Grid allows you to run tests on multiple machines and browsers simultaneously, improving test execution speed by parallelizing tests across different configurations.
Â
24.How do you handle different browsers (cross-browser testing) in Selenium?
Selenium provides browser-specific drivers like ChromeDriver
, FirefoxDriver
, and EdgeDriver
. You can also use Selenium Grid to execute tests on different browsers and machines concurrently.
Â
25.What is TestNG and how does it integrate with Selenium?
TestNG is a testing framework for Java that supports features like parallel test execution, grouping, and data-driven testing. It integrates with Selenium to manage and organize test scripts.
Â
26.What are the challenges you have faced while using Selenium?
Common challenges include handling dynamic elements, synchronizing tests with page loads, working with multiple browser versions, and managing browser drivers in different environments.
Â
27.How do you run Selenium tests in parallel?
Use TestNG’s parallel execution feature or Selenium Grid to run tests on multiple browsers or machines simultaneously to speed up the testing process.
Â
28.How would you implement data-driven testing in Selenium?
Use TestNG’s @DataProvider
to pass different sets of data to the test methods, or use external data sources like Excel or CSV files for data-driven testing.
Â
29.What are some best practices for creating maintainable Selenium scripts?
Use the Page Object Model (POM) for modular code, avoid hardcoded waits, implement logging, and use version control like Git for better test management and collaboration.
Â
30.What is the importance of FluentWait
in Selenium?
FluentWait allows fine-grained control over polling intervals and custom handling of exceptions, making it more flexible for waiting on elements in situations with varying load times.
Â
31.How do you handle SSL certificate errors in Selenium WebDriver?
Configure WebDriver to accept SSL certificates by setting desired capabilities or browser-specific options, such as using DesiredCapabilities
in Chrome and Firefox.